Update README.md
This commit is contained in:
parent
3f79cb5e81
commit
bc9fa1c057
@ -1 +1 @@
|
||||
WIP
|
||||
Check https://ffmpegwasm.netlify.app/docs/contribution/core
|
||||
|
185
README.md
185
README.md
@ -9,11 +9,9 @@
|
||||
[](https://github.com/emersion/stability-badges#experimental)
|
||||
[](https://img.shields.io/node/v/@ffmpeg/ffmpeg.svg)
|
||||
[](https://github.com/ffmpegwasm/ffmpeg.wasm/actions)
|
||||

|
||||

|
||||
[](https://github.com/ffmpegwasm/ffmpeg.wasm/graphs/commit-activity)
|
||||
[](https://opensource.org/licenses/MIT)
|
||||
[](https://github.com/airbnb/javascript)
|
||||
[](https://www.npmjs.com/package/@ffmpeg/ffmpeg)
|
||||
[](https://www.npmjs.com/package/@ffmpeg/ffmpeg)
|
||||
|
||||
@ -23,180 +21,9 @@ Join us on Discord!
|
||||
|
||||
ffmpeg.wasm is a pure Webassembly / Javascript port of FFmpeg. It enables video & audio record, convert and stream right inside browsers.
|
||||
|
||||
**AVI to MP4 Demo**
|
||||
<p align="center">
|
||||
<a href="#">
|
||||
<img alt="transcode-demo" src="https://github.com/ffmpegwasm/ffmpeg.wasm/raw/master/docs/images/transcode.gif">
|
||||
</a>
|
||||
</p>
|
||||
|
||||
Try it: [https://ffmpegwasm.netlify.app](https://ffmpegwasm.netlify.app#demo)
|
||||
|
||||
Check next steps of ffmpeg.wasm [HERE](https://github.com/ffmpegwasm/ffmpeg.wasm/discussions/415)
|
||||
|
||||
## Installation
|
||||
|
||||
**Node**
|
||||
|
||||
```
|
||||
$ npm install @ffmpeg/ffmpeg @ffmpeg/core
|
||||
```
|
||||
|
||||
> As we are using experimental features, you need to add flags to run in Node.js
|
||||
|
||||
```
|
||||
$ node --experimental-wasm-threads transcode.js
|
||||
```
|
||||
|
||||
**Browser**
|
||||
|
||||
Or, using a script tag in the browser (only works in some browsers, see list below):
|
||||
|
||||
> SharedArrayBuffer is only available to pages that are [cross-origin isolated](https://developer.chrome.com/blog/enabling-shared-array-buffer/#cross-origin-isolation). So you need to host [your own server](https://github.com/ffmpegwasm/ffmpegwasm.github.io/blob/main/server/server.js) with `Cross-Origin-Embedder-Policy: require-corp` and `Cross-Origin-Opener-Policy: same-origin` headers to use ffmpeg.wasm.
|
||||
|
||||
```html
|
||||
<script src="static/js/ffmpeg.min.js"></script>
|
||||
<script>
|
||||
const { createFFmpeg } = FFmpeg;
|
||||
...
|
||||
</script>
|
||||
```
|
||||
|
||||
> Only browsers with SharedArrayBuffer support can use ffmpeg.wasm, you can check [HERE](https://caniuse.com/sharedarraybuffer) for the complete list.
|
||||
|
||||
## Usage
|
||||
|
||||
ffmpeg.wasm provides simple to use APIs, to transcode a video you only need few lines of code:
|
||||
|
||||
```javascript
|
||||
const fs = require('fs');
|
||||
const { createFFmpeg, fetchFile } = require('@ffmpeg/ffmpeg');
|
||||
|
||||
const ffmpeg = createFFmpeg({ log: true });
|
||||
|
||||
(async () => {
|
||||
await ffmpeg.load();
|
||||
ffmpeg.FS('writeFile', 'test.avi', await fetchFile('./test.avi'));
|
||||
await ffmpeg.run('-i', 'test.avi', 'test.mp4');
|
||||
await fs.promises.writeFile('./test.mp4', ffmpeg.FS('readFile', 'test.mp4'));
|
||||
process.exit(0);
|
||||
})();
|
||||
```
|
||||
|
||||
### Use other version of ffmpeg.wasm-core / @ffmpeg/core
|
||||
|
||||
For each version of ffmpeg.wasm, there is a default version of @ffmpeg/core (you can find it in **devDependencies** section of [package.json](https://github.com/ffmpegwasm/ffmpeg.wasm/blob/master/package.json)), but sometimes you may need to use newer version of @ffmpeg/core to use the latest/experimental features.
|
||||
|
||||
**Node**
|
||||
|
||||
Just install the specific version you need:
|
||||
|
||||
```bash
|
||||
$ npm install @ffmpeg/core@latest
|
||||
```
|
||||
|
||||
Or use your own version with customized path
|
||||
|
||||
```javascript
|
||||
const ffmpeg = createFFmpeg({
|
||||
corePath: '../../../src/ffmpeg-core.js',
|
||||
});
|
||||
```
|
||||
|
||||
**Browser**
|
||||
|
||||
```javascript
|
||||
const ffmpeg = createFFmpeg({
|
||||
corePath: 'static/js/ffmpeg-core.js',
|
||||
});
|
||||
```
|
||||
|
||||
Keep in mind that for compatibility with webworkers and nodejs this will default to a local path, so it will attempt to look for `'static/js/ffmpeg.core.js'` locally, often resulting in a local resource error. If you wish to use a core version hosted on your own domain, you might reference it relatively like this:
|
||||
|
||||
```javascript
|
||||
const ffmpeg = createFFmpeg({
|
||||
corePath: new URL('static/js/ffmpeg-core.js', document.location).href,
|
||||
});
|
||||
```
|
||||
|
||||
For the list available versions and their changelog, please check: https://github.com/ffmpegwasm/ffmpeg.wasm-core/releases
|
||||
|
||||
### Use single thread version
|
||||
|
||||
```javascript
|
||||
const ffmpeg = createFFmpeg({
|
||||
mainName: 'main',
|
||||
corePath: 'https://unpkg.com/@ffmpeg/core-st@0.11.1/dist/ffmpeg-core.js',
|
||||
});
|
||||
```
|
||||
|
||||
## Multi-threading
|
||||
|
||||
Multi-threading need to be configured per external libraries, only following libraries supports it now:
|
||||
|
||||
### x264
|
||||
|
||||
Run it multi-threading mode by default, no need to pass any arguments.
|
||||
|
||||
### libvpx / webm
|
||||
|
||||
Need to pass `-row-mt 1`, but can only use one thread to help, can speed up around 30%
|
||||
|
||||
## Documentation
|
||||
|
||||
- [API](https://github.com/ffmpegwasm/ffmpeg.wasm/blob/master/docs/api.md)
|
||||
- [Supported External Libraries](https://github.com/ffmpegwasm/ffmpeg.wasm-core#configuration)
|
||||
|
||||
## FAQ
|
||||
|
||||
### What is the license of ffmpeg.wasm?
|
||||
|
||||
There are two components inside ffmpeg.wasm:
|
||||
|
||||
- @ffmpeg/ffmpeg (https://github.com/ffmpegwasm/ffmpeg.wasm)
|
||||
- @ffmpeg/core (https://github.com/ffmpegwasm/ffmpeg.wasm-core)
|
||||
|
||||
@ffmpeg/core contains WebAssembly code which is transpiled from original FFmpeg C code with minor modifications, but overall it still following the same licenses as FFmpeg and its external libraries (as each external libraries might have its own license).
|
||||
|
||||
@ffmpeg/ffmpeg contains kind of a wrapper to handle the complexity of loading core and calling low-level APIs. It is a small code base and under MIT license.
|
||||
|
||||
### Can I use ffmpeg.wasm in Firefox?
|
||||
|
||||
Yes, but only for Firefox 79+ with proper header in both client and server, visit https://ffmpegwasm.netlify.app to try whether your Firefox works.
|
||||
|
||||
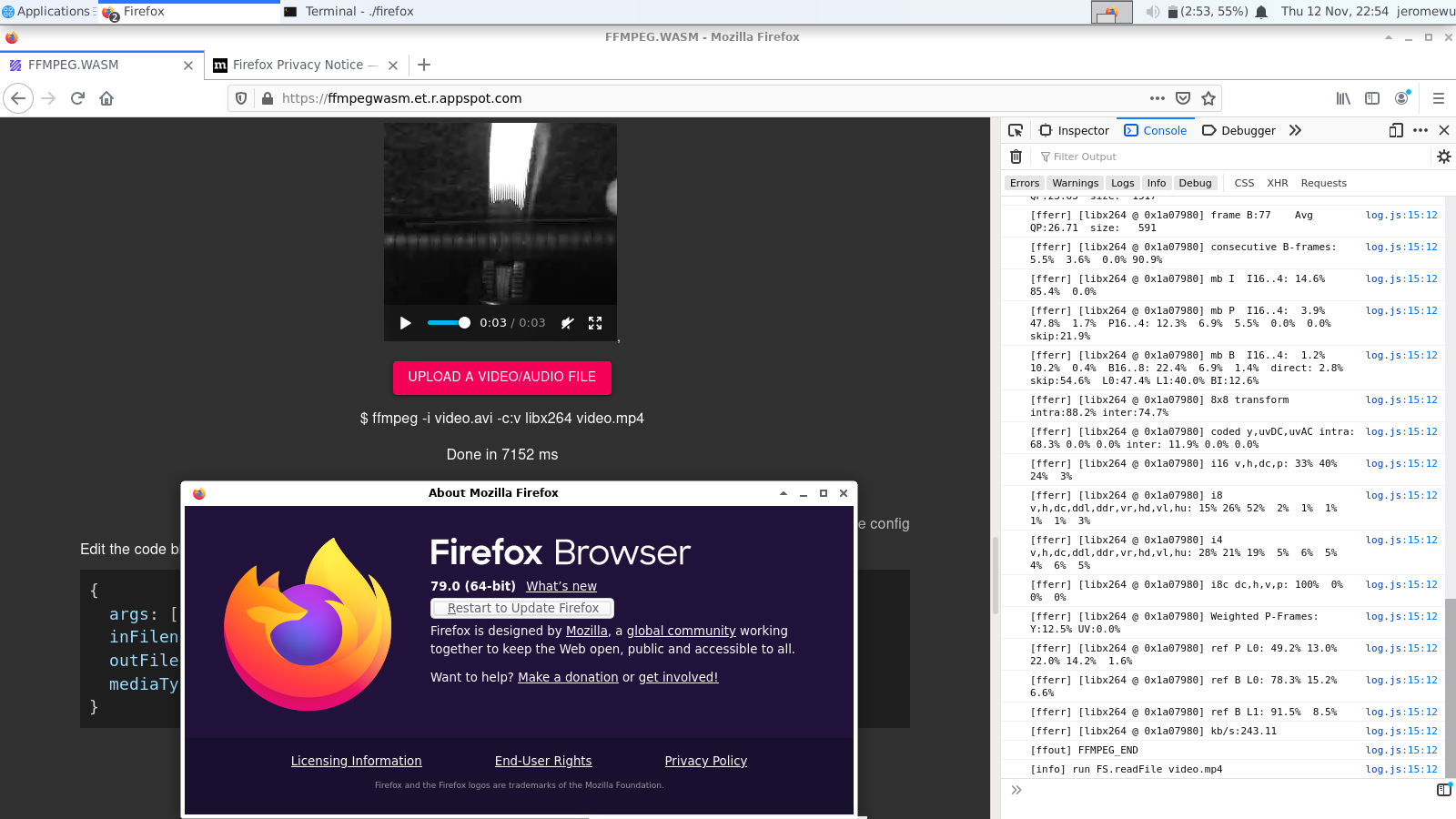
|
||||
|
||||
For more details: https://github.com/ffmpegwasm/ffmpeg.wasm/issues/106
|
||||
|
||||
### What is the maximum size of input file?
|
||||
|
||||
2 GB, which is a hard limit in WebAssembly. Might become 4 GB in the future.
|
||||
|
||||
### How can I build my own ffmpeg.wasm?
|
||||
|
||||
In fact, it is ffmpeg.wasm-core most people would like to build.
|
||||
|
||||
To build on your own, you can check build.sh inside https://github.com/ffmpegwasm/ffmpeg.wasm-core repository.
|
||||
|
||||
Also you can check this series of posts to learn more fundamental concepts:
|
||||
|
||||
- https://jeromewu.github.io/build-ffmpeg-webassembly-version-part-1-preparation/
|
||||
- https://jeromewu.github.io/build-ffmpeg-webassembly-version-part-2-compile-with-emscripten/
|
||||
- https://jeromewu.github.io/build-ffmpeg-webassembly-version-part-3-v0.1/
|
||||
- https://jeromewu.github.io/build-ffmpeg-webassembly-version-part-4-v0.2/
|
||||
|
||||
### Why it doesn't work in my local environment?
|
||||
|
||||
When calling `ffmpeg.load()`, by default it looks for `http://localhost:3000/node_modules/@ffmpeg/core/dist/` to download essential files (ffmpeg-core.js, ffmpeg-core.wasm, ffmpeg-core.worker.js). It is necessary to make sure you have those files served there.
|
||||
|
||||
If you have those files serving in other location, you can rewrite the default behavior when calling `createFFmpeg()`:
|
||||
|
||||
```javascript
|
||||
const { createFFmpeg } = FFmpeg;
|
||||
const ffmpeg = createFFmpeg({
|
||||
corePath: "http://localhost:3000/public/ffmpeg-core.js",
|
||||
// Use public address if you don't want to host your own.
|
||||
// corePath: 'https://unpkg.com/@ffmpeg/core@0.10.0/dist/ffmpeg-core.js'
|
||||
log: true,
|
||||
});
|
||||
```
|
||||
- [Introduction](https://ffmpegwasm.netlify.app/docs/intro)
|
||||
- [Getting
|
||||
Started](https://ffmpegwasm.netlify.app/docs/getting-started/installation)
|
||||
- [API](https://ffmpegwasm.netlify.app/docs/api/classes/FFmpeg)
|
||||
- [FAQ](https://ffmpegwasm.netlify.app/docs/faq)
|
||||
- [Contribution](https://ffmpegwasm.netlify.app/contribution/core)
|
||||
|
@ -1 +1,30 @@
|
||||
# FAQ
|
||||
|
||||
### What is the license of ffmpeg.wasm?
|
||||
|
||||
There are two components inside ffmpeg.wasm:
|
||||
|
||||
- @ffmpeg/ffmpeg (https://github.com/ffmpegwasm/ffmpeg.wasm)
|
||||
- @ffmpeg/core (https://github.com/ffmpegwasm/ffmpeg.wasm-core)
|
||||
|
||||
@ffmpeg/core contains WebAssembly code which is transpiled from original FFmpeg C code with minor modifications, but overall it still following the same licenses as FFmpeg and its external libraries (as each external libraries might have its own license).
|
||||
|
||||
@ffmpeg/ffmpeg contains kind of a wrapper to handle the complexity of loading core and calling low-level APIs. It is a small code base and under MIT license.
|
||||
|
||||
### What is the maximum size of input file?
|
||||
|
||||
2 GB, which is a hard limit in WebAssembly. Might become 4 GB in the future.
|
||||
|
||||
### How can I build my own ffmpeg.wasm?
|
||||
|
||||
In fact, it is `@ffmpeg/core` most people would like to build.
|
||||
|
||||
To build on your own, you can check [Contribution Guide](/docs/contribution/core)
|
||||
|
||||
Also you can check this series of posts to learn more fundamental concepts
|
||||
(OUTDATED, but still good to learn foundations):
|
||||
|
||||
- https://jeromewu.github.io/build-ffmpeg-webassembly-version-part-1-preparation/
|
||||
- https://jeromewu.github.io/build-ffmpeg-webassembly-version-part-2-compile-with-emscripten/
|
||||
- https://jeromewu.github.io/build-ffmpeg-webassembly-version-part-3-v0.1/
|
||||
- https://jeromewu.github.io/build-ffmpeg-webassembly-version-part-4-v0.2/
|
||||
|
205
docs/api.md
205
docs/api.md
@ -1,205 +0,0 @@
|
||||
# API
|
||||
|
||||
- [createFFmpeg()](#create-ffmpeg)
|
||||
- [ffmpeg.load()](#ffmpeg-load)
|
||||
- [ffmpeg.run()](#ffmpeg-run)
|
||||
- [ffmpeg.FS()](#ffmpeg-fs)
|
||||
- [ffmpeg.exit()](#ffmpeg-exit)
|
||||
- [ffmpeg.setLogging()](#ffmpeg-setlogging)
|
||||
- [ffmpeg.setLogger()](#ffmpeg-setlogger)
|
||||
- [ffmpeg.setProgress()](#ffmpeg-setProgress)
|
||||
- [fetchFile()](#fetch-file)
|
||||
|
||||
---
|
||||
|
||||
<a name="create-ffmpeg"></a>
|
||||
|
||||
## createFFmpeg(options): ffmpeg
|
||||
|
||||
createFFmpeg is a factory function that creates a ffmpeg instance.
|
||||
|
||||
**Arguments:**
|
||||
|
||||
- `options` an object of customized options
|
||||
- `corePath` path for ffmpeg-core.js script
|
||||
- `log` a boolean to turn on all logs, default is `false`
|
||||
- `logger` a function to get log messages, a quick example is `({ message }) => console.log(message)`
|
||||
- `progress` a function to trace the progress, a quick example is `p => console.log(p)`
|
||||
|
||||
**Examples:**
|
||||
|
||||
```javascript
|
||||
const { createFFmpeg } = FFmpeg;
|
||||
const ffmpeg = createFFmpeg({
|
||||
corePath: "./node_modules/@ffmpeg/core/dist/ffmpeg-core.js",
|
||||
log: true,
|
||||
});
|
||||
```
|
||||
|
||||
<a name="ffmpeg-load"></a>
|
||||
|
||||
### ffmpeg.load(): Promise
|
||||
|
||||
Load ffmpeg.wasm-core script.
|
||||
|
||||
In browser environment, the ffmpeg.wasm-core script is fetch from CDN and can be assign to a local path by assigning `corePath`. In node environment, we use dynamic require and the default `corePath` is `$ffmpeg/core`.
|
||||
|
||||
Typically the load() func might take few seconds to minutes to complete, better to do it as early as possible.
|
||||
|
||||
**Examples:**
|
||||
|
||||
```javascript
|
||||
(async () => {
|
||||
await ffmpeg.load();
|
||||
})();
|
||||
```
|
||||
|
||||
<a name="ffmpeg-run"></a>
|
||||
|
||||
### ffmpeg.run(...args): Promise
|
||||
|
||||
This is the major function in ffmpeg.wasm, you can just imagine it as ffmpeg native cli and what you need to pass is the same.
|
||||
|
||||
**Arguments:**
|
||||
|
||||
- `args` string arguments just like cli tool.
|
||||
|
||||
**Examples:**
|
||||
|
||||
```javascript
|
||||
(async () => {
|
||||
await ffmpeg.run('-i', 'flame.avi', '-s', '1920x1080', 'output.mp4');
|
||||
/* equals to `$ ffmpeg -i flame.avi -s 1920x1080 output.mp4` */
|
||||
})();
|
||||
```
|
||||
|
||||
<a name="ffmpeg-fs"></a>
|
||||
|
||||
### ffmpeg.FS(method, ...args): any
|
||||
|
||||
Run FS operations.
|
||||
|
||||
For input/output file of ffmpeg.wasm, it is required to save them to MEMFS first so that ffmpeg.wasm is able to consume them. Here we rely on the FS methods provided by Emscripten.
|
||||
|
||||
For more info, check https://emscripten.org/docs/api_reference/Filesystem-API.html
|
||||
|
||||
**Arguments:**
|
||||
|
||||
- `method` string method name
|
||||
- `args` arguments to pass to method
|
||||
|
||||
**Examples:**
|
||||
|
||||
```javascript
|
||||
/* Write data to MEMFS, need to use Uint8Array for binary data */
|
||||
ffmpeg.FS('writeFile', 'video.avi', new Uint8Array(...));
|
||||
/* Read data from MEMFS */
|
||||
ffmpeg.FS('readFile', 'video.mp4');
|
||||
/* Delete file in MEMFS */
|
||||
ffmpeg.FS('unlink', 'video.mp4');
|
||||
```
|
||||
|
||||
<a name="ffmpeg-exit"></a>
|
||||
|
||||
### ffmpeg.exit()
|
||||
|
||||
Kill the execution of the program, also remove MEMFS to free memory
|
||||
|
||||
**Examples:**
|
||||
|
||||
```javascript
|
||||
const ffmpeg = createFFmpeg({ log: true });
|
||||
await ffmpeg.load(...);
|
||||
setTimeout(() => {
|
||||
ffmpeg.exit(); // ffmpeg.exit() is callable only after load() stage.
|
||||
}, 1000);
|
||||
await ffmpeg.run(...);
|
||||
```
|
||||
|
||||
<a name="ffmpeg-setlogging"></a>
|
||||
|
||||
### ffmpeg.setLogging(logging)
|
||||
|
||||
Control whether to output log information to console
|
||||
|
||||
**Arguments**
|
||||
|
||||
- `logging` a boolean to turn of/off log messages in console
|
||||
|
||||
**Examples:**
|
||||
|
||||
```javascript
|
||||
ffmpeg.setLogging(true);
|
||||
```
|
||||
|
||||
<a name="ffmpeg-setlogger"></a>
|
||||
|
||||
### ffmpeg.setLogger(logger)
|
||||
|
||||
Set customer logger to get ffmpeg.wasm output messages.
|
||||
|
||||
**Arguments**
|
||||
|
||||
- `logger` a function to handle the messages
|
||||
|
||||
**Examples:**
|
||||
|
||||
```javascript
|
||||
ffmpeg.setLogger(({ type, message }) => {
|
||||
console.log(type, message);
|
||||
/*
|
||||
* type can be one of following:
|
||||
*
|
||||
* info: internal workflow debug messages
|
||||
* fferr: ffmpeg native stderr output
|
||||
* ffout: ffmpeg native stdout output
|
||||
*/
|
||||
});
|
||||
```
|
||||
|
||||
<a name="ffmpeg-setprogress"></a>
|
||||
|
||||
### ffmpeg.setProgress(progress)
|
||||
|
||||
Progress handler to get current progress of ffmpeg command.
|
||||
|
||||
**Arguments**
|
||||
|
||||
- `progress` a function to handle progress info
|
||||
|
||||
**Examples:**
|
||||
|
||||
```javascript
|
||||
|
||||
ffmpeg.setProgress(({ ratio }) => {
|
||||
console.log(ratio);
|
||||
/*
|
||||
* ratio is a float number between 0 to 1.
|
||||
*/
|
||||
});
|
||||
```
|
||||
|
||||
<a name="fetch-file"></a>
|
||||
|
||||
### fetchFile(media): Promise
|
||||
|
||||
Helper function for fetching files from various resource.
|
||||
|
||||
Sometimes the video/audio file you want to process may located in a remote URL and somewhere in your local file system.
|
||||
|
||||
This helper function helps you to fetch to file and return an Uint8Array variable for ffmpeg.wasm to consume.
|
||||
|
||||
**Arguments**
|
||||
|
||||
- `media` an URL string, base64 string or File, Blob, Buffer object
|
||||
|
||||
**Examples:**
|
||||
|
||||
```javascript
|
||||
(async () => {
|
||||
const data = await fetchFile('https://github.com/ffmpegwasm/testdata/raw/master/video-3s.avi');
|
||||
/*
|
||||
* data will be in Uint8Array format
|
||||
*/
|
||||
})();
|
||||
```
|
Binary file not shown.
Before Width: | Height: | Size: 10 KiB |
Binary file not shown.
Before Width: | Height: | Size: 839 KiB |
Loading…
Reference in New Issue
Block a user